Unity3D: Display a Social Share Dialog for Android Games
Android Intents allow developers to share data such as text, images and URLs with other apps. This is handy for “Share” buttons, allowing users to tweet, post, email or message their friends from within your app or game.
For games in particular, this is extremely useful for letting users invite their friends to play, share their high scores, or show off their latest achievements.
In case you’re unfamiliar, here’s what the share dialog looks like on Android devices. As you can see, the user is able to select whichever app they like to share to, and once an app is selected they can tweak and personalize the content as they see fit.
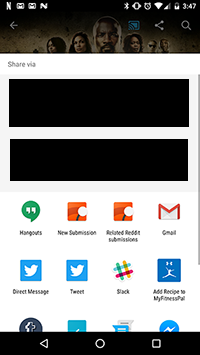
Note that I had to block out some personal contact information, but the user’s frequent contacts will be displayed in the top portion for Email, SMS, and Messaging.
Implementing an Intent in Java is rather straightforward and a very common requirement for all kinds of tasks:
Intent intent = new Intent(); intent.setAction(Intent.ACTION_SEND); intent.putExtra(Intent.EXTRA_TEXT, "Here's the text I want to share."); intent.setType("text/plain"); startActivity(Intent.createChooser(intent));
These few lines of code provide you with the ability to share to a limitless number of apps, and can be a powerful way to let your users promote your game!
Sharing with Unity
Unity doesn’t provide the functionality required for this out-of-the-box, however it does provide us with the AndroidJavaClass and AndroidJavaObject classes. These two classes provide all the functionality we need to interface with Java code on Android devices, giving us the ability to create our Intent.
The first thing we need to do is to get a reference to two Java classes that we need, one being the Intent class, and the second is the UnityPlayer class, both of which we’ll need to construct and send our Intent.
// Get the required Intent and UnityPlayer classes. AndroidJavaClass intentClass = new AndroidJavaClass("android.content.Intent"); AndroidJavaClass unityPlayerClass = new AndroidJavaClass("com.unity3d.player.UnityPlayer");
By providing the fully qualified Java class name to the AndroidJavaClass constructor, we get a reference to the Java class which we can use to write the full Intent logic.
Next we’ll construct the Intent just like the Java code above.
// Construct the intent. AndroidJavaObject intent = new AndroidJavaObject("android.content.Intent"); intent.Call<AndroidJavaObject>("setAction", intentClass.GetStatic<string>("ACTION_SEND")); intent.Call<AndroidJavaObject>("putExtra", intentClass.GetStatic<string>("EXTRA_TEXT"), "Here's the text I want to share."); intent.Call<AndroidJavaObject>("setType", "text/plain");
The AndroidJavaObject constructor also takes the fully qualified Java class name, but rather than giving us the class, it gives us an Intent instance. This is equivalent to the Intent intent = new Intent(); Java code from above.
Next we call the setAction, putExtra and setType methods on the Intent instance, just like we did in Java. We use the intentClass reference to get the static ACTION_SEND and EXTRA_TEXT properties, which we got directly using Intent.ACTION_SEND and Intent.EXTRA_TEXT in Java. We pass the string we want to share when setting the EXTRA_TEXT, and finally set the Intent type to text/plain.
Finally, with the Intent constructed and initialized, it’s time to display the chooser dialog. We use the UnityPlayer class reference from above, unityPlayerClass, to get the currently displayed Activity. We call the static createChooser method on the Intent class, and provide it with our Intent instance. This wraps the Intent in another Intent that forces the chooser dialog to be displayed, preventing a default app from automatically opening if the user has one set. See Intent.createChooser if you want more details on how that works.
The very last set is to call startActivity on the currentActivity, passing in our wrapped chooser Intent, to display the chooser dialog, and from there the user will be able to select their preferred app to share your message!
// Display the chooser. AndroidJavaObject currentActivity = unityPlayerClass.GetStatic<AndroidJavaObject>("currentActivity"); AndroidJavaObject chooser = intentClass.CallStatic<AndroidJavaObject>("createChooser", intent, "Share"); currentActivity.Call("startActivity", chooser);
As you can see, interfacing with Java code is a little clunky from C#, but it’s really quite simple once you get used to it.
Binding the Click Event
At this point, we’re ready to bind the click event of the “Share” button to our code above. Here’s the full function, called OnShareClicked. The only difference here is we explicitly check that we’re running on an Android device using the UNITY_ANDROID directive. This is useful if you’re targeting multiple platforms, for instance iOS.
public void OnShareClicked() { #if UNITY_ANDROID // Get the required Intent and UnityPlayer classes. AndroidJavaClass intentClass = new AndroidJavaClass("android.content.Intent"); AndroidJavaClass unityPlayerClass = new AndroidJavaClass("com.unity3d.player.UnityPlayer"); // Construct the intent. AndroidJavaObject intent = new AndroidJavaObject("android.content.Intent"); intent.Call<AndroidJavaObject>("setAction", intentClass.GetStatic<string>("ACTION_SEND")); intent.Call<AndroidJavaObject>("putExtra", intentClass.GetStatic<string>("EXTRA_TEXT"), "Here's the text I want to share."); intent.Call<AndroidJavaObject>("setType", "text/plain"); // Display the chooser. AndroidJavaObject currentActivity = unityPlayerClass.GetStatic<AndroidJavaObject>("currentActivity"); AndroidJavaObject chooser = intentClass.CallStatic<AndroidJavaObject>("createChooser", intent, "Share"); currentActivity.Call("startActivity", chooser); #endif }
In the Unity editor, simply bind your button’s OnClick listener to the OnShareClicked function:
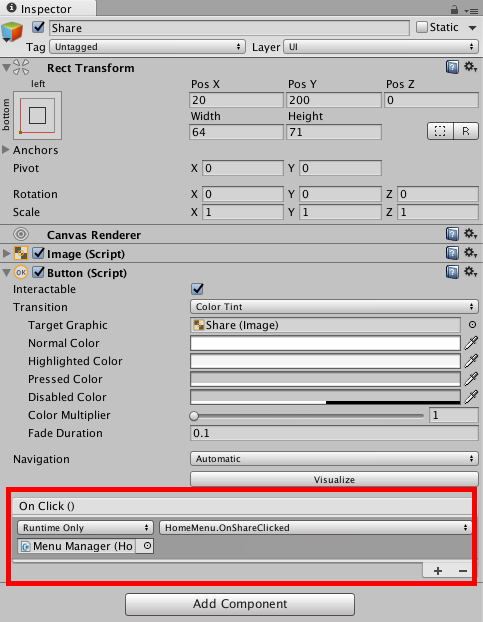
And you’re all set! Run your game on an Android device and give the button a click to test it out.