Customizing (or Disabling) the Global Gravity in Unity
Gravity is an important component of many 2D and 3D games, and understanding how to tweak gravity either for the entire game, or on-the-fly depending on game state, can help you create realistic (or wildly unrealistic) environments for your players to explore.
Unity provides a standard gravity simulation located in the Physics and Physics2D classes for 3D and 2D Unity games, respectively. The gravity values in these packages can be accessed using Physics.gravity and Physics2D.gravity depending on your environment, and are represented by a Vector3 for 3D games, and a Vector2 for 2D games.
By default, Unity provides games with the following values:
// Physics2D.gravity x: 0.0, y: -9.8 // Physics.gravity x: 0.0, y: -9.8, z: 0.0
As you can see, both provide a default - 9.8 on the y-axis. This simulates the gravity we’re accustomed to by pulling objects down, but that may or may not be what you require for your game.
These gravity values can be globally changed so that all objects in your game behave in a particular way. For instance, you may want them to be pulled up, to the left or right, or just modify the gravitational force to make it stronger or weaker. You may even want to disable it altogether.
Luckily the value can be changed quite simply and on-the-fly.
Disable Gravity
To disable gravity you would simply do the following:
// 2D Physics2D.gravity = Vector2.zero; // 3D Physics.gravity = Vector3.zero;
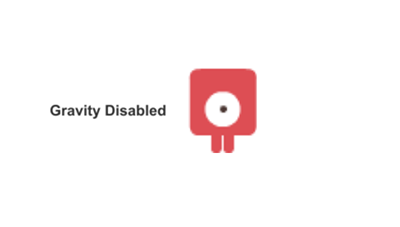
By setting the gravitational force to zero, it effectively removes any pull on your game objects so they are free to move around in any direction without gravitational pull.
Set Custom Gravity
You can also set custom gravity values. For instance, the following will pull game objects up and to the right:
Physics2D.gravity = new Vector2(10.0f, 10.0f);
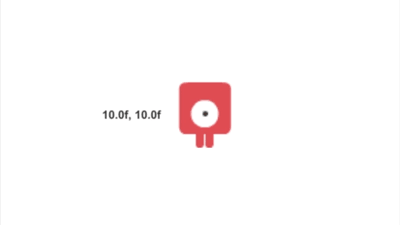
Or you can simply increase or decrease the force of the standard gravity:
// Four times as strong Physics2D.gravity = new Vector2(0, Physics2D.gravity.y * 4); // Half the strength Physics2D.gravity = new Vector2(0, Physics2D.gravity.y / 2);
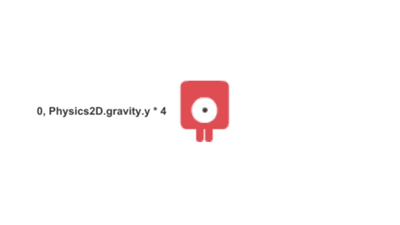
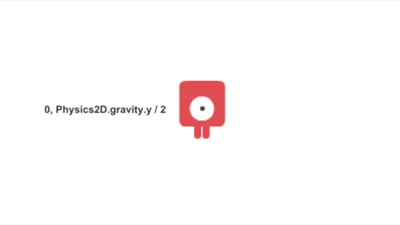
This allows you to customize gravity however you see fit to suit your game. A game that takes place in space may want to disable gravity for instance, while a platforming game may want to inverse gravity for a particular level or even on-the-fly with a power-up to walk on the ceiling.
However you need gravity to behave in your 2D or 3D game, its as easy as a single line of code to make it happen.
Sample Project
I’ve created a sample project on GitHub that allows you to easily play around with custom gravity values using the sprite from the images in this post.
The project includes a Gravity Manager object on the main scene, that contains input for custom gravity values. You can also check the source code in CustomGravity.cs to see the contents of this post in action!