Drawing Triangles, Rhombuses and Other Custom Shapes on an Android Canvas
The Android Canvas provides convenient methods for drawing simple shapes such as circles and rectangles, using drawCircle and drawRect respectively, but beyond these the majority of shapes require some custom Path logic to draw. Two shapes that I was required to draw were triangles and rhombuses, so I thought I’d share how I accomplished this.
As I mentioned above, the Paths can be used in conjunction with the drawPath method to draw any shapes or patterns you like. Usage is pretty straightforward, you just have to provide drawPath with a Path and Paint object and let it do the drawing:
Path path = ...; Paint paint = ...; canvas.drawPath(path, paint);
But how do you actually construct a path for a triangle or rhombus?
Triangles
Let’s start with the triangle as it’s a slightly simpler shape than a rhombus. The way a Path works is by drawing lines between points, so we’ll need to pick a point as the starting position, and draw lines to each vertex. As you’ll see in the code below, we’ll be starting from the top vertex and moving counter-clockwise around the triangle to each additional vertex:
public void drawTriangle(Canvas canvas, Paint paint, int x, int y, int width) { int halfWidth = width / 2; Path path = new Path(); path.moveTo(x, y - halfWidth); // Top path.lineTo(x - halfWidth, y + halfWidth); // Bottom left path.lineTo(x + halfWidth, y + halfWidth); // Bottom right path.lineTo(x, y - halfWidth); // Back to Top path.close(); canvas.drawPath(path, paint); }
Simply call drawTriangle with the Canvas to draw on, the Paint to draw with, the X/Y coordinates to draw at, and the width of the triangle.
Let’s define a Paint object and see how it turns out:
Paint paint = new Paint(); paint.setColor(context.getColor(android.R.color.black)); drawTriangle(canvas, paint, 100, 100, 100);
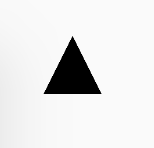
Not bad, with a little customization such as modifying the color or applying antialiasing this can work quite well for your triangle drawing needs.
Rhombus
Drawing a rhombus follows the same principle, just with an extra vertex. As you can see below, the Path is almost identical, just with the addition of a single extra vertex for the bottom of the rhombus:
public void drawRhombus(Canvas canvas, Paint paint, int x, int y, int width) { int halfWidth = width / 2; Path path = new Path(); path.moveTo(x, y + halfWidth); // Top path.lineTo(x - halfWidth, y); // Left path.lineTo(x, y - halfWidth); // Bottom path.lineTo(x + halfWidth, y); // Right path.lineTo(x, y + halfWidth); // Back to Top path.close(); canvas.drawPath(path, paint); }
Given the same Paint as above, we can now draw the rhombus:
Paint paint = new Paint(); paint.setColor(context.getColor(android.R.color.black)); drawTriangle(canvas, paint, 100, 100, 100); drawRhombus(canvas, paint, 100, 300, 50);
And we should see a rhombus on the view like so:
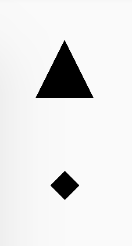
Other Shapes
Given you now know how to draw triangles and rhombuses using custom paths, drawing other shapes such as hexagons or octagons is simply a matter of adjusting the Path to form the shape you need. Additionally, the examples above assume a standard triangle and rhombus shape, but adjusting to say, a right-angle triangle, can be accomplished by modifying the Path as well.