Easier Programmatic 2D and 3D Unity Animations with Unimation
Unimation is an open source project I’ve been developing that aims to make animations in Unity 2D and 3D projects easier by providing access to various flexible and efficient animations.
I frequently find myself writing animation scripts that would scale, move, and rotate GameObjects (with a slight variance on the previous time I’d written a similar script), and decided it was time to create a library that I could drop into any Unity project and hit the ground running.
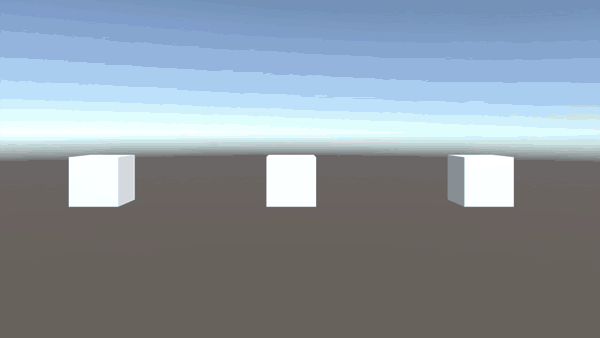
Animations
There are currently three animations supported in Unimation with more on the way:
- ScaleTo: Animates the scale of the GameObject.
- MoveTo: Animates the position of the GameObject.
- RotateTo: Animates the rotation of the GameObject.
There are two additional variations of ScaleTo to accommodate common use cases - scaling a GameObject from zero to one, and scaling a GameObject from one to zero:
- ScaleIn: Animates the scale of the GameObject to Vector3.one.
- ScaleOut: Animates the scale of the GameObject to Vector3.zero.
Customization
The goal of Unimation is to provide flexible customization options that can handle various situations, regardless of what the project requires.
In order to support this goal, Unimation currently supports the following customization:
- duration Required: The time, in seconds, that the animation should take.
- interpolation Optional: Defines how the animation should progress over time. Linear, accelerate, decelerate are currently supported/planned, with more to come as needed.
- looping Optional: Allows you to loop the animation a set number of times, or infinitely.
Examples
All of the animations can be either statically run using Unimation.Animate or by extending Unimation.Behaviour instead of MonoBehaviour and calling methods on your custom scripts:
// Static ... Unimation.Animate.ScaleTo(gameObject, scale, duration); // ... or Subclassing public class MyScript : Unimation.Behaviour { void Start() { this.ScaleTo(scale, duration); } }
The GIF at the top of the post demonstrating the three animating cubes was created using the following scripts:
ScaleToSample.cs
public class ScaleToSample : Unimation.Behaviour { void Start() { ScaleTo(Vector3.zero, 3.0f, Unimation.Mode.Linear, Unimation.Animation.LoopInfinite); } }
MoveToSample.cs
public class MoveToSample : Unimation.Behaviour { void Start() { MoveTo(Vector3.one, 3.0f, Unimation.Mode.Linear, Unimation.Animation.LoopInfinite); } }
RotateToSample.cs
public class RotateToSample : Unimation.Behaviour { void Start() { RotateTo(new Vector3(45f, 45f, 0f), 3.0f, Unimation.Mode.Linear, Unimation.Animation.LoopInfinite); } }
For more examples, and for installation and usage guides, check out the Unimation repository on GitHub.
Roadmap and Contributing
My first order of business is to finish the accelerate and decelerate interpolators as time permits, and to add support for animating the color of SpriteRenderers, mostly for alpha animation purposes.
Additionally, I plan to provide support for more customization and animations as needed, and would appreciate contributions from the Unity community to the GitHub Repository if there’s anything you’d like to add!