Animating Android Activities and Views with Left and Right Slide Animations
Animations, when used correctly, can be a simple way to enhance the user experience of your products, adding a little bit of fun that a motionless view just doesn’t have. Today I’ll be demonstrating how to add some basic left and right sliding animations to your Views and Activities on Android.
First, here’s a look at the animation we’ll be creating. As you can see, the activities slide in and out of view based on the navigation direction appropriate for the transition:
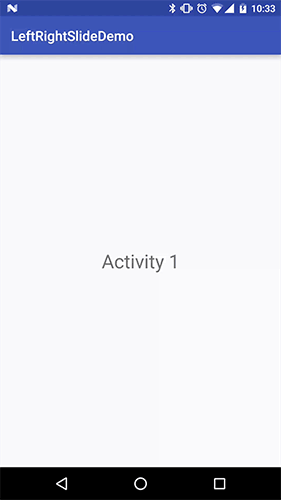
Defining the Animations
For our purposes, we’ll need four animations in total, and we’ll be defining them through XML. Of the four animations, there are really two groups. The first is to move a view from its current position to a position out of view, and the second is to bring a view in from out of view.
slide_to_left.xml:
Animates from the current position, all the way to the left and out of view.
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="0" android:toXDelta="-100%p" android:interpolator="@android:anim/accelerate_decelerate_interpolator" android:duration="@integer/slide_animation_duration"/> </set>
slide_to_right.xml:
Animates from the current position, all the way to the right and out of view.
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="0" android:toXDelta="100%p" android:interpolator="@android:anim/accelerate_decelerate_interpolator" android:duration="@integer/slide_animation_duration"/> </set>
slide_from_left.xml:
Animates from out of view on the left, into view.
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="-100%p" android:toXDelta="0" android:interpolator="@android:anim/accelerate_decelerate_interpolator" android:duration="@integer/slide_animation_duration"/> </set>
slide_from_right.xml:
Animates from out of view on the right, into view.
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="100%p" android:toXDelta="0" android:interpolator="@android:anim/accelerate_decelerate_interpolator" android:duration="@integer/slide_animation_duration"/> </set>
Each of the animations has a duration value of @integer/slide_animation_duration, which is defined in integers.xml like so:
<?xml version="1.0" encoding="utf-8"?> <resources> <integer name="slide_animation_duration">300</integer> </resources>
This helps to keep consistency between all animations, and ensures that they all move at the same speed.
On a View
Animating a single View is straightforward, we just load the animation from the XML and apply it to the view:
view.startAnimation(AnimationUtils.loadAnimation( context, R.anim.slide_to_right ));
I prefer to create a helper class for these groups of related animations, especially if they are going to be common within the application:
public class SlideAnimationUtil { /** * Animates a view so that it slides in from the left of it's container. * * @param context * @param view */ public static void slideInFromLeft(Context context, View view) { runSimpleAnimation(context, view, R.anim.slide_from_left); } /** * Animates a view so that it slides from its current position, out of view to the left. * * @param context * @param view */ public static void slideOutToLeft(Context context, View view) { runSimpleAnimation(context, view, R.anim.slide_to_left); } /** * Animates a view so that it slides in the from the right of it's container. * * @param context * @param view */ public static void slideInFromRight(Context context, View view) { runSimpleAnimation(context, view, R.anim.slide_from_right); } /** * Animates a view so that it slides from its current position, out of view to the right. * * @param context * @param view */ public static void slideOutToRight(Context context, View view) { runSimpleAnimation(context, view, R.anim.slide_to_right); } /** * Runs a simple animation on a View with no extra parameters. * * @param context * @param view * @param animationId */ private static void runSimpleAnimation(Context context, View view, int animationId) { view.startAnimation(AnimationUtils.loadAnimation( context, animationId )); } }
After this helper class is created, we can use it from anywhere in our application like so:
SlideAnimationUtil.slideInFromLeft(context, view);
Between Activities
Just as easy is animating the transition between Activities. The Activity class provides us with a method called overridePendingTransition that we can use to set the animation of the exiting and entering Activities, like so:
Intent intent = new Intent(this, SomeActivity.class); startActivity(intent); overridePendingTransition(enterAnimationId, exitAnimationId);
Calling overridePendingTransition after the call to startActivity will ensure that the newly started Activity runs the enterAnimationId and the current Activity runs the exitAnimationId.
Using the animations we defined earlier, we can have the new Activity slide in from the right of the view, and the current Activity slide out of view to the left, like so:
startActivity(intent); overridePendingTransition(R.anim.slide_from_right, R.anim.slide_to_left);
Similarly, when the new Activity is finished, we can perform the reverse animation to have the finished Activity slide out of view to the right, and the previous Activity slide back into view from the left:
finish(); overridePendingTransition(R.anim.slide_from_left, R.anim.slide_to_right);
This gives a smooth transition back and forth between the Activities that may be preferable to you than the default Activity transition animation.
Keeping Consistency
In most of my applications I create a BaseActivity class that all my Activities extend, and put common functionality shared by all in there, such as logging and displaying fragments for example. Since I generally like to have all Activity transitions to be consistent throughout the app, I find this to be a perfect candidate for the BaseActivity:
public class BaseActivity extends Activity { @Override public void finish() { super.finish(); overridePendingTransitionExit(); } @Override public void startActivity(Intent intent) { super.startActivity(intent); overridePendingTransitionEnter(); } /** * Overrides the pending Activity transition by performing the "Enter" animation. */ protected void overridePendingTransitionEnter() { overridePendingTransition(R.anim.slide_from_right, R.anim.slide_to_left); } /** * Overrides the pending Activity transition by performing the "Exit" animation. */ protected void overridePendingTransitionExit() { overridePendingTransition(R.anim.slide_from_left, R.anim.slide_to_right); } }
You can also go so far as to override the startActivity and finish methods to directly call these two methods as well, so that your Activities never need to worry about calling them directly. Just be sure that you always call the super method, and to override all variations such as startActivityForResult.
Now you can be sure that if you decide to change your transition animations, you only have to update them in one place, and that all of your Activities are animating consistently.
Bonus: Handling the Back Button
Note that this isn’t applicable if you override the finish method, because finish will eventually be called anyways when the back button is pressed.
The animation when finishing your Activity above is great when you call finish directly, but what if the user clicks the device’s back button to finish the Activity? Simply override the onBackPressed method and add your transition:
@Override public void onBackPressed() { super.onBackPressed(); overridePendingTransitionExit(); }
Bonus: Animating Toolbar and TabLayout Background Colors
Another slick touch you can add your applications is animating the background color of your Toolbar and TabLayout!